(This is a follow-up to my previous post on the topic. To see all the examples in a slightly more readable format, click here.)
Magic Item Type Example
Here we have the classic Magic Item Type selection table from page 23 of Monsters & Treasure.
The Code for Magic Item Type
This script selects items from a table that has one result for a range of rolls. The list syntax is slightly different than we've seen. It's a list that contains lists.
<script> function getMagicItemType() { var magicItemTypesTable = [ [20, "Swords"], [15, "Armor"], [5, "Misc. Weapons"], [25, "Potions"], [20, "Scrolls"], [5, "Rings"], [5, "Wands/Staves"], [5, "Misc. Magic"] ]; function randomTableValue(table) { // How large is this table? What's the maximum roll? d12, d100, or what? var max = 0; for (var i = 0; i < table.length; i++) { max = max + table[i][0]; } var roll = Math.floor(Math.random() * max) + 1; // Find our roll result in the table: var countUpToRoll = 0; for (var i = 0; i < table.length; i++) { if (roll <= table[i][0] + countUpToRoll) { return "d" + max + " = " + roll + ": " + table[i][1]; } else { countUpToRoll = countUpToRoll + table[i][0]; } } } var itemType = document.getElementById("magicitemtype"); itemType.innerHTML = randomTableValue(magicItemTypesTable); } </script> <div> <button onclick="getMagicItemType();">Roll Magic Item Type</button> <span id="magicitemtype"></span> </div>
Arena Fighter Name Generator
We can slightly vary the generated content with randomness and conditional execution.
Math.random()
returns a value between 0 and 1.
<script> function arenaFighterName() { function arnd(a) { // Return random element of array a. var i = Math.floor(Math.random() * (a.length)); if (typeof a[i] === 'function') { return a[i](); } return a[i]; } function namePart1() { return arnd([ "Al", "En", "Ro", "Fel", "Ston", "Hal", "Jo", "Nel", "Ve", "Ga" ]) + arnd([ "rick", "bert", "wick", "thor", "ky", "son", "frey", "sley", "gil" ]); } function namePart2() { var x = Math.random(); if (x < 0.25) { return "the " + arnd([ "Brave", "Bloody", "Bold", "Cruel", "Clever", "Cunning", "Indomitable", "Destroyer", "Quick", "Heartless", "Sly" ]); } else if (x < 0.5) { return "of " + arnd([ "Green", "New", "River", "Dun", "Lun", "North", "Wolver", "Tam", "Chapel", "Wit", "Bran", "Mor", "Ep", "Grims", "Gos" ]) + arnd([ "thorpe", "by", "ford", "bury", "ham", "shire", "ton", "don", "ly", "field", "beck", "gate", "well", "holme", "wick", "port" ]); } else if (x < 0.75) { return namePart1(); } else { return ""; } } var name = namePart1() + " " + namePart2(); var outputSpan = document.getElementById("arenafightername"); outputSpan.innerHTML = name; } </script> <div> <button onclick="arenaFighterName();">Arena Fighter Name</button> <span id="arenafightername"></span> </div>
Dynamically Chosen and Arranged Images (Geomorphs!)
We can play with images as well as text.
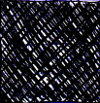
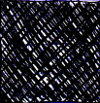
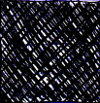
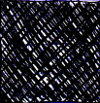
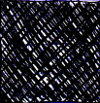
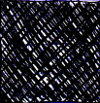
<script> function shuffleGeomorphs() { function arnd(a) { // Return random element of array a. var i = Math.floor(Math.random() * (a.length)); if (typeof a[i] === 'function') { return a[i](); } return a[i]; } var geomorphImages = [ "img/g01.png", "img/g02.png", "img/g03.png", "img/g04.png", "img/g05.png", "img/g06.png", "img/g07.png", "img/g08.png", ]; var imageOutputs = document.getElementById('geomorphgrid').childNodes; for(var i = 0; i < imageOutputs.length; i++) { imageOutputs[i].src = arnd(geomorphImages); } } </script> <button onclick="shuffleGeomorphs();">Shuffle Geomorphs</button> <div id="geomorphgrid"> <img src="img/g00.png"> <img src="img/g00.png"> <img src="img/g00.png"> <br> <img src="img/g00.png"> <img src="img/g00.png"> <img src="img/g00.png"> </div>
Great examples again.
ReplyDeleteHow and where are the geomorphs stored before they are shown?
The image files would have to be stored on the web at a know URL, so the actual items in the geomorphImages array might look like "https://farm6.staticflickr.com/5306/5669546361_c08f1517d8_s.jpg".
ReplyDeleteokay thanks, should have realized but for some reason my brain wasn't clicking on it correctly.
Delete